top of page
Projects
ICOM 4075 - Advanced Programming
Project #1 - Snake
In this project I worked to implement the following features:
​
-
Changed color of the snake segments.
-
Changed the Background Color to purple using the Class “Color” and RGB values instead of color constants.
-
Changed grid size to 60x60.
-
Changed the speed of the snake.
-
Implemented the keyboard button ‘N’ so that when pressed you can add a piece of tail.
-
Implemented the keyboard button ‘+’ (‘-’) so that when pressed you can increase(decrease) the snake speed respectively.
-
Implemented a score.
-
Every dot the snake eats will increase the score by the square root of two times the current score plus one.)
-
Display a “Game Over” dialog screen when the snake collides with itself.
-
Made the snake teleport to the opposite end (relative to the direction going) when colliding with a wall.
-
Prevented Backtracking, in other words, do not allow the snake to move back over itself.
-
Made the game be able to pause at the touch of the ‘ESC’ key.
-
Modified the apple class to have a property called isGood() that will tell if the apple is still good or rotten. If good, the apple will behave as it does. If it's rotten, the apple should change to a different color so that it looks rotten and if it's eaten, the score should be decreased by the square root of two times the current score plus one and the snake should lose a tail.
-
The good apples get rotten if the player has not eaten them after x amount of ‘steps’, where a step is the snake moving from one square to the next and x = grid width.
​
Other features:
-
Apart from the required features, I also implemented a credit. The player gets credit from grabbing coins and the credit number does not go down if you eat a rotten apple as the score does. The coins work just like the apples do, but they appear at a smaller rate.
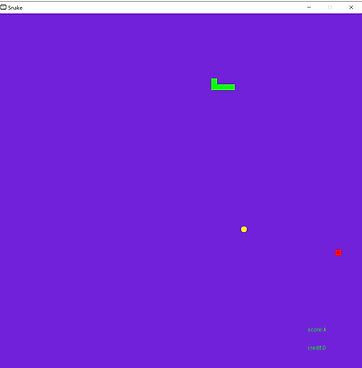_edited.jpg)
Project #2 - Dinner Dash
In this project, I worked with my teammate Andrea del Campillo and these were some of the features we implemented:
​
Basic features:
​
-
Added other objects to the restaurant, not used before. (We added TVs, the jukebox and napkins.)
-
Created a new type of Counter with peppers that replaced an empty counter.
-
Implemented the "shift" key to make the player move slower.
Patience and payment features:
​
-
If a client is served before his patience reaches half, he should pay a tip of 15% of the amount paid for the burger.
-
Getting any order exactly right should give all the clients a fourth of their patience back.
-
Instead of checking all clients to see which order matches, had the chef be able to serve any specific customer by pressing numbers one through 5 (correspondent to his position in the line) in front of the Plate Counter.
-
Added a new counter that sets a random amount of ticks (in the thousands), when this amount of ticks has passed, the counter will change color for 2 seconds, if interacted in that time all customer’s patience will be maxed out.
-
Made it so that if the burger's tint is between 0.48 and 0.53, the player will get an extra 12% of what the client was going to give the player.
​
Clients:
​
-
Created a special client called “inspector”. If he doesn’t get his food in time, the player will lose half his money earned, and all future customers have 6% less patience due to a bad review, but getting it on time will increase everyone’s patience by 12%, and all future clients have 10% more patience.
-
Created a special client called “Anti-V”. As their patience goes down, every 8% will lower another client's that is in front or behind (randomly) patience by 4%.
-
I made the images for these characters using Photoshop and editing some of the other character's images.
​
Win or Lose:
​
-
Made it so when the player wins $50, he wins. Winning will send you to a WinState, from where you can restart, or go to the main menu.
-
Made the game tracks how many people he served, and how many left and display it in the WinState and in the GameState.
-
Made it so if 10 people leave, you lose and get sent to a LoseState which displays the same things WinState does plus the amount of money made.
​
Other features:
​
-
Apart from the required features assigned in this project, I also implemented drinks so that the clients can order soda or water for $1 each. I made a soda/water counter where the player can select soda by pressing the keyboard 'S' or water with the 'W'. They can serve it as they serve the burger. To be able to understand this, I added the controls menu to the main menu which indicates the functions of each keyboard.
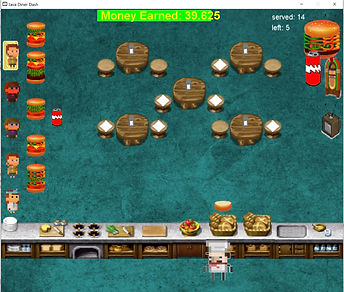_edited.jpg)
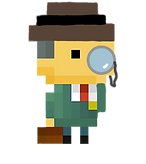
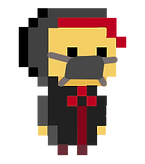
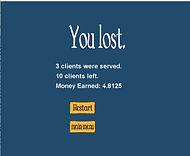_edited.jpg)
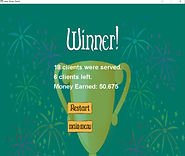_edited.jpg)
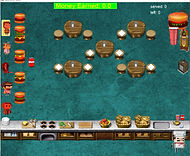_edited.jpg)
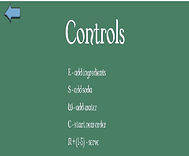
Project #3 - Minecraft Mod
In this project I worked with a partner Ana Laura to create a Minecraft mod that included the following features:
Items:
-
Pizza item
-
Pizza Cake
-
Skull block
-
Rainbow Ore which generates in the world randomly.
-
Armor: Pizza helmet, pizza chestplate, pizza leggings, and pizza boots.
-
Weapon: Pizza sword.
-
Tools: Pizza axe, pizza pickaxe, pizza hoe, and pizza shovel.
​
I made these items images in photoshop (with the exception of the skull block) by editing already existing Minecraft items. For example, I used the gold armor from Minecraft, to create my pizza armor's texture.
​
Recipes:
Smelting:
-
Rainbow Ore → Pizza item
Shapeless:
-
9 pizza items → 1 skull block
-
1 skull block → 9 pizza items
-
1 pizza item + 1 cake → Pizza Cake
-
1 repeater + 1 bow → Laser Gun
-
1 comparator + chest → Charge Pack
Shaped:
-
Armor recipes (shaped like the original armor ones in Minecraft) for the pizza helmet, pizza chestplate, pizza leggings, and pizza boots.
-
Weapon recipe (shaped like the original one in Minecraft) for the pizza sword.
-
Tools recipes (shaped like the original tool ones in Minecraft) for the pizza axe, pizza pickaxe, pizza hoe, and pizza shovel.
​
Beam Entity:
​
-
Implemented a beam entity that is based on the arrow. This entity will despawn when walked on, so the player never picks up a beam like he picks up an arrow when it lands on the floor.
​
Laser Gun:
​
-
Implemented a Laser gun item based on the bow item. It has all the basic functionalities of a bow, but instead of arrows, it’ll look for the charge pack and consume some of its durability to shoot the beam entity.
-
The laser gun has and uses durability like a regular bow and all its attributes, but its durability is higher than a normal bow's.
-
The laser gun’s draw speed (the speed before it can fire a beam) is faster than a regular bow and will have higher damage than the bow.
-
If on creative, the laser gun will shoot no matter if you have a charge pack on you or not.
​
​
Charge Pack:​
​
-
Implemented a Charge pack item that does not break and has a stack size of 1.
-
It’ll have max durability. When right-clicked, it will search the player's inventory for Redstone dust or Redstone blocks. If it finds one, it’ll consume one and raise its durability by two different amounts depending on if its the dust or the block. If durability is maxed, it will not raise it anymore nor look in the inventory for the Redstone items.
-
The charge pack displays how much charge it has in the tooltip.
-
The charge pack will never go below 1 charge.
-
If it has 1 charge, it’ll display 0 on the tooltip and it won’t shoot.
​
I made the textures for the laser gun, the charge pack and the beam using photoshop.​











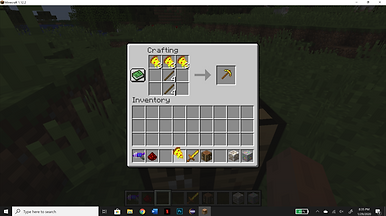.png)
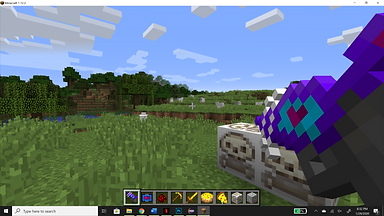.png)







bottom of page